Mastering Maven: A Comprehensive Guide to Java Dependency Management and Build Automation
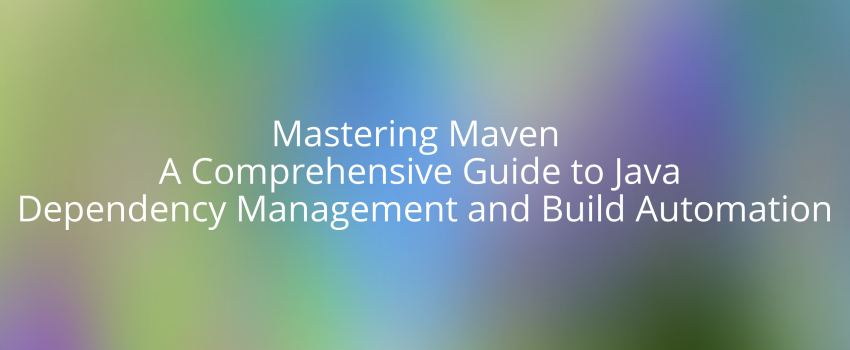
Introduction to Maven: What is it and Why Do We Use it?
Maven is a popular build automation tool used primarily for Java projects. It simplifies the build process and manages dependencies of a project, making it easier to develop and maintain Java applications. Maven is an open-source tool developed by Apache Software Foundation.
One of the key benefits of using Maven is that it allows developers to manage project dependencies easily. Maven automatically downloads the required dependencies from the Maven repository, a centralized location for storing project dependencies. This eliminates the need for developers to manually download and manage dependencies.
Managing Dependencies in Java: The Manual Way vs. Using Maven
If you are developing a Java application, chances are you will need to use some external libraries or frameworks that provide functionality that you don't want to write yourself. These libraries or frameworks are called dependencies, and they can make your life easier by saving you time and effort. However, managing dependencies can also be a challenge, especially if you have many of them or if they have their own dependencies.
One way to manage dependencies is to do it manually. This means that you have to download the jar files of the dependencies you need, and add them to your project's classpath. You also have to keep track of the versions of the dependencies you use, and update them when necessary. This can be tedious and error-prone, especially if you have to deal with transitive dependencies (dependencies of dependencies) or conflicts between different versions of the same dependency.
Another way to manage dependencies is to use a tool like Maven. Maven is a software project management and comprehension tool that can help you automate the process of managing dependencies. With Maven, you don't have to download the jar files of the dependencies yourself; instead, you specify them in a file called pom.xml, which is the main configuration file for your project. Maven will then download the dependencies for you from a central repository, and add them to your project's classpath. Maven will also handle transitive dependencies and version conflicts for you, by using a mechanism called dependency mediation.
Maven has many other features besides dependency management, such as project building, testing, reporting, documentation, and deployment. However, in this blog post, we will focus on how Maven can help you manage dependencies in Java more easily and effectively than doing it manually.
Getting Started with Maven: How to Create a Maven Project
Maven is a popular tool for managing Java projects. It helps you automate tasks such as compiling, testing, packaging, and deploying your code. In this blog post, we will show you how to create a simple Maven project from scratch.
First, you need to install Maven on your computer. You can download it from https://maven.apache.org/download.cgi and follow the installation instructions. You also need to have Java installed and set up the JAVA_HOME environment variable.
Next, you need to create a directory for your project and open a terminal or command prompt in that directory. Then, you can use the following command to generate a basic project structure using a Maven archetype:
mvn -B archetype:generate -DgroupId=com.example -DartifactId=my-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command will create a directory named my-app with the following files and folders:
my-app
|-- pom.xml
`-- src
|-- main
| `-- java
| `-- com
| `-- example
| `-- App.java
`-- test
`-- java
`-- com
`-- example
`-- AppTest.java
The pom.xml file is the core of your Maven project. It contains information about your project and its dependencies. The src/main/java folder contains your source code, and the src/test/java folder contains your test code.
The App.java file is a simple Java class that prints "Hello World!" to the standard output. The AppTest.java file is a unit test class that uses JUnit to verify the behavior of the App class.
To build your project, you can use the following command:
mvn package
This command will compile your code, run your tests, and create a JAR file named my-app-1.0-SNAPSHOT.jar in the target folder.
To run your project, you can use the following command:
java -cp target/my-app-1.0-SNAPSHOT.jar com.example.App
This command will execute the main method of the App class and print "Hello World!" to the console.
Congratulations! You have created your first Maven project. You can now explore more features of Maven, such as adding dependencies, plugins, profiles, etc. You can also use an IDE such as Eclipse or IntelliJ IDEA to work with your Maven project.
Maven Tutorial Video
In the video, Kunal explains the role of Maven in automating your project build process, from the compilation of source code to running unit and integration tests for your application, and packaging your application into a jar or war which you will finally deploy. He shares a story about a developer named Rahul who struggled with manually managing dependencies for his large Java project, but then discovered Maven, a build automation tool that promised to simplify his work.
With Maven, Rahul was able to manage dependencies using a structured document called a POM, which kept track of all the libraries used in the project and their respective versions. Not only did Maven make it easy to manage dependencies, but it also streamlined the entire life cycle of the project, from building and compiling to testing, packaging, and deploying. This allowed Rahul to focus more on writing code and less on managing dependencies, ultimately making his life easier.
Kunal also covers key elements of Maven, including POM, Dependencies, Goals, Plugins, Build lifecycle, Profile, and Repositories. He explains how Maven manages dependencies for you and shows you how to create a new Maven project using Archetype templates, which are pre-defined templates that provide all the required file and directory structures for you. Kunal also provides a detailed explanation of the installation and setup process for Maven, as well as how to publish your library/jar to Maven Central Repo and others can use it.
So, whether you're a beginner or an experienced Java developer, this video on Maven is a must-watch. You'll learn the ins and outs of Maven, how to manage dependencies, streamline your project life cycle, and more. With Kunal's easy-to-understand explanations and examples, you'll be able to start using Maven in no time. So, what are you waiting for? Watch the video today and start mastering Maven!
Understanding the POM: A Closer Look at the Project Object Model
If you are using Maven to build your Java projects, you might have encountered a file named pom.xml in your project directory. This file is called the POM, which stands for Project Object Model. It is an XML file that contains information about your project and how Maven should build it. In this blog post, we will take a closer look at the POM and its elements.
The POM is the fundamental unit of work in Maven. It defines the project's structure, dependencies, build process, reporting, and more. It also inherits default values and configurations from a Super POM, which is Maven's default POM. You can override or customize these values in your own POM.
The POM has a hierarchical structure, where each element can have sub-elements. The root element is <project>, which must have a <modelVersion> element that specifies the POM version (currently 4.0.0). The <project> element must also have three mandatory elements: <groupId>, <artifactId>, and <version>. These elements form the project's coordinates, which uniquely identify your project in Maven's repository.
The <groupId> element defines the group or organization that owns the project, such as com.example or org.apache. The <artifactId> element defines the name of the project, such as my-app or commons-lang. The <version> element defines the version of the project, such as 1.0 or 2.5-SNAPSHOT. Together, these elements form a fully qualified artifact name, such as com.example:my-app:1.0.
The <packaging> element defines the type of artifact that Maven will produce from your project, such as jar, war, ear, or pom. If you omit this element, Maven will use jar as the default packaging type.
The <dependencies> element defines the external libraries or modules that your project depends on, such as junit, log4j, or spring-core. Each dependency is specified by a <dependency> element that contains its coordinates and optionally other attributes, such as scope, classifier, or exclusions.
The <parent> element defines a parent POM that your project inherits from, such as a corporate or framework POM. This allows you to share common configurations and properties across multiple projects. The parent POM must also have its coordinates specified by a <groupId>, <artifactId>, and <version> element.
The <dependencyManagement> element defines a set of dependencies that can be used by submodules or child projects without specifying their versions or other attributes. This allows you to centralize and manage dependency versions in one place.
The <modules> element defines a set of submodules or child projects that are part of a larger project, such as a multi-module project or an aggregator project. Each submodule is specified by a <module> element that contains its relative path to its directory.
The <properties> element defines a set of key-value pairs that can be used to configure other elements in the POM or in plugins. For example, you can define a property for the Java source version and use it in the compiler plugin configuration.
The POM also contains other elements that provide more information about your project and its environment, such as:
XML tag | Description |
---|---|
<build> |
Defines how Maven should build your project, including plugins, goals, profiles, resources, etc. |
<profiles> |
Allows you to define different configurations for different environments. |
<reporting> |
Defines how Maven should generate reports from your project, such as test results, code coverage, javadoc, etc. |
<name> |
Defines a human-readable name for your project. |
<description> |
Defines a brief description of your project. |
<url> |
Defines a URL where your project can be accessed or downloaded. |
<inceptionYear> |
Defines the year when your project was started. |
<licenses> |
Defines the licenses that apply to your project. |
<organization> |
Defines the organization that owns or sponsors your project. |
<developers> |
Defines the developers who are involved in your project. |
<contributors> |
Defines the contributors who have helped with your project. |
<issueManagement> |
Defines how issues or bugs are tracked and managed for your project. |
<ciManagement> |
Defines how continuous integration is performed for your project. |
<mailingLists> |
Defines the mailing lists where discussions about your project take place. |
<scm> |
Defines how source code management is done for your project, and allows you to define different configurations for different environments. |
<pluginRepositories> |
Specifies the repositories from which plugins should be resolved. |
Maven Artifacts: Packaging, Publishing, and Sharing Your Code
Maven is a popular tool for managing Java projects and their dependencies. One of the key features of Maven is that it can produce artifacts, which are files that contain the compiled code, resources, and metadata of a project. Artifacts can be used by other projects as dependencies, or they can be published to repositories for sharing and distribution.
In this blog post, we will explore how Maven handles artifacts, what are the different types of packaging, how to publish artifacts to various repositories, and how to share them with other developers.
Packaging Types
The packaging type of a Maven project determines what kind of artifact it produces. Maven supports several default packaging types, such as jar, war, ear, pom, rar, ejb, and maven-plugin. Each packaging type has a specific format and structure, and follows a different build lifecycle with different goals.
For example, a jar packaging type produces a Java archive file with the .jar extension, which contains Java classes, interfaces, resources, and metadata files. A war packaging type produces a web application archive file with the .war extension, which contains web components such as servlets, JSPs, HTML pages, and resources.
To specify the packaging type of a Maven project, we need to add the <packaging> element in the pom.xml file. For example:
<packaging>jar</packaging>
If no packaging type is specified, Maven assumes it is a jar by default.
Publishing Artifacts
Maven allows us to publish artifacts to various repositories for sharing and distribution. A repository is a place where artifacts are stored and accessed by other projects or tools. There are two types of repositories: local and remote.
A local repository is a directory on our machine where Maven stores all the artifacts that it downloads or builds. By default, it is located at ~/.m2/repository. We can use the mvn install command to install our artifacts to the local repository.
A remote repository is a server that hosts artifacts and makes them available over a network. There are many public remote repositories that host open-source artifacts, such as Maven Central or JCenter. We can also use private remote repositories for our own projects or organizations, such as GitHub Package Registry or Azure Artifacts.
To publish our artifacts to a remote repository, we need to configure the <distributionManagement> element in the pom.xml file with the repository information. For example:
<distributionManagement>
<repository>
<id>my-repo</id>
<url>https://my-repo.com/maven</url>
</repository>
</distributionManagement>
We also need to provide authentication credentials for the remote repository in the settings.xml file located at ~/.m2/settings.xml. For example:
<servers>
<server>
<id>my-repo</id>
<username>my-username</username>
<password>my-password</password>
</server>
</servers>
Then we can use the mvn deploy command to publish our artifacts to the remote repository.
Sharing Artifacts
Once we have published our artifacts to a remote repository, we can share them with other developers or projects by adding them as dependencies. A dependency is a reference to an artifact that our project needs to compile, test, or run.
To add a dependency to our project, we need to specify the groupId, artifactId, and version of the artifact in the <dependencies> element in the pom.xml file. For example:
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>my-artifact</artifactId>
<version>1.0.0</version>
</dependency>
</dependencies>
Maven will automatically download the artifact from the remote repository and store it in the local repository. It will also resolve any transitive dependencies that the artifact may have.
Maven Repositories: Local vs. Remote, Public vs. Private
If you are a Java developer, you probably have used Maven to manage your project dependencies and build process. Maven is a powerful tool that simplifies the development workflow and automates many tasks. One of the key features of Maven is its repository system, which allows you to store and retrieve artifacts (such as jars, wars, poms, etc.) from different sources.
But what are the types of repositories that Maven supports and how do they differ? In this blog post, we will explore the four main categories of Maven repositories: local vs. remote, and public vs. private.
Local Repository
A local repository is a directory on your machine that stores the artifacts that you have downloaded or installed. By default, it is located at ~/.m2/repository, but you can change it in your settings.xml file. The local repository acts as a cache for the artifacts that you need for your projects. It also allows you to work offline without accessing any remote repositories.
Remote Repository
A remote repository is a repository that is hosted on a server and accessible via a URL. It can be either public or private, depending on the access permissions. A remote repository can host artifacts that are not available in your local repository or in any other repositories that you have configured. You can specify the remote repositories that you want to use in your pom.xml file or in your settings.xml file.
Public Repository
A public repository is a remote repository that is open to anyone on the internet. It can host artifacts that are widely used by the Java community, such as libraries, frameworks, plugins, etc. The most popular public repository is Maven Central, which is maintained by Sonatype and contains over 2 million artifacts. You can also use other public repositories, such as JCenter, Google Cloud Platform, or GitHub Packages.
Private Repository
A private repository is a remote repository that is restricted to a specific group of users or organizations. It can host artifacts that are proprietary or confidential, such as internal libraries, custom plugins, or enterprise applications. You can set up your own private repository using tools like Nexus, Artifactory, or Archiva. You can also use cloud-based services like Azure DevOps Services or AWS CodeArtifact.
Build Automation with Maven: Streamlining the Project Life Cycle
If you are a Java developer, you probably have heard of Maven, a powerful tool for managing and building Java projects. But what exactly is Maven and how can it help you streamline your project life cycle?
Maven is based on the concept of a project object model (POM), which is an XML file that contains information about the project and its configuration, such as dependencies, plugins, goals, etc. Maven uses this file to perform various tasks, such as compiling, testing, packaging, and deploying your code.
Maven also simplifies the process of managing project dependencies, ensuring that you always use the correct versions of libraries and frameworks in your project. Maven can automatically download and install dependencies from a central repository, or you can specify your own custom repositories.
Maven supports a standard project structure, which makes it easy for developers to understand and navigate the project. Maven also provides a common interface for building software, using plugins that can be retrieved from the Maven repository. Plugins can add additional functionality to the build process, such as code coverage analysis, static code analysis, and more.
Maven defines a build life cycle, which consists of a sequence of phases that are executed in order. Each phase consists of one or more goals, which are specific tasks that Maven performs. For example, the compile phase has a goal to compile the source code, the test phase has a goal to run unit tests, and so on.
By using Maven, you can automate and standardize your build process, making it more consistent and reliable. You can also generate various reports and documentation from your source code, such as Javadoc, test results, dependency lists, etc.
Maven is a great tool for streamlining your project life cycle and making your work easier and more efficient.
Installing Maven: A Step-by-Step Guide
I have created blog post on Maven installation steps
Beginner's Guide to Installing and Setting up Maven | PDI Blog (parvatdharainnovations.com)
Maven Archetypes: Using Pre-Defined Templates to Create New Projects
Hello, fellow Maven enthusiasts! Today I'm going to show you how to use pre-defined templates to create new projects in a snap. These templates are called Maven Archetypes, and they are awesome!
What is a Maven Archetype, you ask? Well, according to the official documentation, "An archetype is defined as an original pattern or model from which all other things of the same kind are made." In other words, it's a project template that you can customize with your own parameters and preferences.
Why would you want to use a Maven Archetype? Because it saves you time and hassle, that's why! Instead of creating a new project from scratch, you can use an existing archetype that matches your needs and goals. For example, if you want to create a simple Java application, you can use the maven-archetype-quickstart archetype. If you want to create a web application, you can use the maven-archetype-webapp archetype. And if you want to create a Maven plugin, you can use the maven-archetype-plugin archetype. There are many more archetypes available for different types of projects, and you can even create your own archetypes if you feel adventurous.
How do you use a Maven Archetype? It's easy! All you need is Maven installed on your machine and a command line interface. Then, you can run the following command:
mvn archetype:generate
This will launch an interactive mode where you can choose an archetype from a list of available ones, or enter a specific archetype groupId, artifactId, and version. You can also specify some properties for your new project, such as groupId, artifactId, version, package, name, description, etc.
Once you have entered all the required information, Maven will generate your new project based on the chosen archetype and place it in a directory with the same name as your artifactId. You can then open it with your favorite IDE or editor and start coding!
That's it! You have just created a new project using a Maven Archetype. Isn't that cool? I hope you enjoyed this tutorial and learned something new.
Advanced Maven: Plugins, Profiles, and Customization
Maven is a powerful tool for managing software projects. It can automate tasks such as compiling, testing, packaging, and deploying your code. But did you know that you can also customize Maven to suit your specific needs? In this blog post, we will explore some of the advanced features of Maven that allow you to create plugins, profiles, and custom settings for your project.
Plugins are extensions that add functionality to Maven. They can perform actions before, during, or after the build process. For example, you can use plugins to generate documentation, run code analysis, or deploy your application to a server. Maven has many built-in plugins that you can use out of the box, but you can also create your own plugins or use third-party ones.
Profiles are configurations that enable or disable certain features based on conditions. They can help you manage different environments, such as development, testing, or production. For example, you can use profiles to specify different dependencies, properties, or plugins for each environment. You can activate profiles by using command-line arguments, environment variables, or files.
Customization is the process of modifying Maven's default behavior or settings. You can customize Maven by using properties, filters, resources, or settings files. For example, you can use properties to define variables that can be used throughout your project. You can use filters to replace placeholders in your files with values from properties. You can use resources to include or exclude files from your project. You can use settings files to configure global or user-specific options for Maven.
By using plugins, profiles, and customization, you can make Maven work for you and your project. You can enhance Maven's capabilities, adapt it to different scenarios, and optimize it for performance and quality. If you want to learn more about these advanced features of Maven, check out the official documentation or some of the online tutorials available.