Understanding Dependency Injection in Java: A Beginner’s Guide
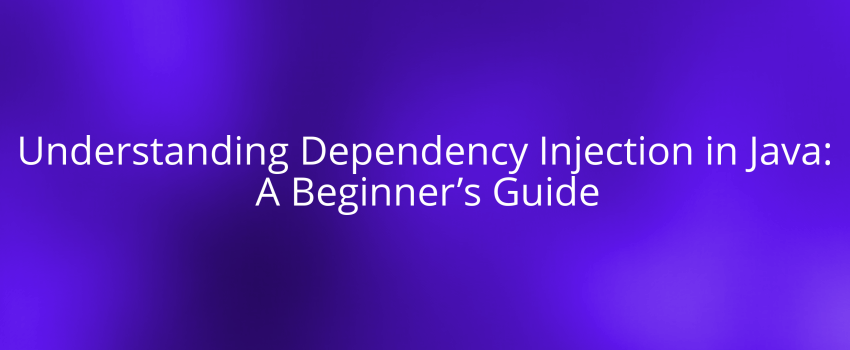
Dependency injection is a powerful technique for decoupling your code components in software development and one of the most used design patterns in Java. It helps you to reduce tight coupling between your application components, allowing them to be more maintainable and testable over time.
With dependency injection, developers can create loosely coupled applications that perform better, enhance scalability, and increase reusability. In this beginner's guide, you will learn more about what dependency injection is, different types of dependency injection, and how to use it in Java. By the end, you'll have the knowledge to create better applications with less hard-coded relationships between classes and components.
Table Of Content.
- Introduction to Dependency Injection
- Types of Dependency Injection
- Frameworks for Dependency Injection
- Dependency Injection Implementation
- Dependency Injection Annotations
- Constructor Injection vs Setter Injection
- Field Injection vs Constructor Injection
- Advanced Dependency Injection Techniques
1- Introduction to Dependency Injection
Dependency Injection (DI) is a technique used in software development that allows the application to manage its dependencies more effectively. Instead of hard-coding dependencies into the code, DI enables them to be defined externally and injected into the application as needed.
Put simply, DI is the process of injecting dependencies into Java classes at runtime, rather than at compile-time. This process is known as inversion of control (IoC), where the control of creating objects is transferred from the application to an external framework.
One of the key advantages of DI is that it makes the code more maintainable and testable. By removing hard-coded dependencies, the code becomes more flexible and easier to modify. Additionally, DI enables unit testing to be done more easily, since the dependencies can be mocked or stubbed.
There are two main types of DI: constructor injection and setter injection. Constructor injection requires that the dependencies be injected through the constructor of the class, while setter injection requires that the dependencies be injected through setter methods.
Here's an example of constructor injection in Java:
public class Car {
private Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
}
In this example, the `Car` class has a dependency on the `Engine` class. Rather than hard-coding the dependency into the class, the dependency is injected through the constructor.
Hard-coded dependencies can lead to code that is tightly coupled, making it difficult to modify and maintain. By using DI, dependencies can be easily changed or updated without the need to modify the code that uses them, making the application more scalable and flexible.
💡 key Takeaway: Dependency Injection is a technique used in software development that allows the application to manage its dependencies more effectively. It enables dependencies to be defined externally and injected into the application as needed, making the code more maintainable, testable, and scalable.
2- Types of Dependency Injection
Now that we have a basic understanding of what dependency injection is and why it is used in Java, it’s time to explore the different types of dependency injection. There are mainly three types of dependency injection: constructor injection, setter method injection, and field injection.
1. Constructor Injection
Constructor injection is the most common type of dependency injection. In this type, dependencies are passed to the class through a constructor. The dependent class defines one or more constructors, and the required dependencies are passed as arguments to the constructor. The following example shows constructor injection:
public class EmployeeService {
private EmployeeDAO employeeDAO;
public EmployeeService(EmployeeDAO employeeDAO) {
this.employeeDAO = employeeDAO;
}
}
As you can see, the dependent class `EmployeeService` takes `EmployeeDAO` as a constructor argument. With this implementation, the `employeeDAO` is instantiated when an object of `EmployeeService` is created.
2. Setter Method Injection
Setter method injection is another type of dependency injection. In this type, dependencies are set through setter methods. The dependent class declares a setter method for a particular dependency that is to be injected. The following example shows setter method injection:
public class EmployeeService {
private EmployeeDAO employeeDAO;
public void setEmployeeDAO(EmployeeDAO employeeDAO) {
this.employeeDAO = employeeDAO;
}
}
As you can see, we have a setter method `setEmployeeDAO()` which sets the `employeeDAO` attribute of the class. With this implementation, the dependency `employeeDAO` can be injected at any point after the object of the class `EmployeeService` is created.
3. Field Injection
Field injection is the least recommended type of dependency injection. In this type, dependencies are injected directly into the class fields. The `@Autowired` annotation in Spring is used for field injection. The following example shows field injection:
public class EmployeeService {
@Autowired
private EmployeeDAO employeeDAO;
}
As you can see, the `employeeDAO` dependency is directly injected into the field of `EmployeeService` class using `@Autowired` annotation.
It is recommended to use constructor or setter method injection instead of field injection because field injection can sometimes cause the application to become unstable, especially in large and complex applications.
💡 key Takeaway: There are mainly three types of dependency injection: constructor injection, setter method injection,
3- Frameworks for Dependency Injection
3 Frameworks for Dependency Injection
Java developers have different options to choose from when it comes to Dependency Injection frameworks. Here are some of the most popular ones:
1. Google Guice
Google Guice is a lightweight, open-source dependency injection framework that simplifies the implementation of complex dependencies in Java applications. It offers an easy-to-use and flexible API that makes dependency injection a breeze. With Guice, developers can reduce code complexity and increase maintainability while following best practices in software design.
2. Spring framework
Spring is a popular framework for building Java-based enterprise applications. It supports several different types of dependency injection, including constructor injection, property injection, and setter injection. Spring is a mature and stable framework, with a large community of developers and an extensive documentation.
3. Java EE
Java EE (Enterprise Edition) is a collection of Java APIs and technologies that are designed for enterprise applications. Java EE includes built-in support for dependency injection through CDI (Contexts and Dependency Injection) which is a Java programming model that allows developers to manage object dependencies within a Java EE application.
Each framework has its own strengths and weaknesses, and the choice between them largely depends on the specific needs of the developer and the project.
(List): Here’s a quick comparison of some features of the three dependency injection frameworks:
Google Guice: lightweight, flexible, easier to learn, great for code maintainability.
Spring framework: full-featured, complete enterprise solution, extensive documentation, mature and stable framework.
Java EE: built-in support through CDI, mature and stable framework, good for enterprise applications.
(Quote): “Choosing the right dependency injection framework for your Java software development project is key to building robust, maintainable and scalable applications.”
💡 key Takeaway: There are several popular dependency injection frameworks for Java, each with its own strengths and weaknesses. The choice of framework depends on the specific needs of the project and the level of support needed for enterprise applications.
4- Dependency Injection Implementation
In Java, dependency injection (DI) is implemented using either the constructor injection or the setter injection approach. Both approaches enable the separation of the creation of an object from its use, providing flexibility in changing an object's dependencies at runtime.
Constructor Injection
As the name suggests, constructor injection involves passing dependencies to a class through its constructor. The following example demonstrates how to create an instance of a UserService class by injecting a UserRepository dependency into its constructor.
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
This approach promotes the "do-it-in-the-constructor" good practice, where a class declares its dependencies through its constructor, making it easy to create testable and modular code.
Setter Injection
Setter injection, on the other hand, involves injecting dependencies to a class through its setter methods. Let's look at an example:
public class UserService {
private UserRepository userRepository;
public UserService() {}
public void setUserRepository(UserRepository userRepository) {
this.userRepository = userRepository;
}
}
In this approach, you first create an instance of the object and then set its dependencies using the available setter methods. Although this approach is more flexible and allows for easier reusability and configuration, it violates the "one-constructor principle," which recommends a single constructor that initializes all the required dependencies of a class.
💡 key Takeaway: In Java, you can implement dependency injection using either the constructor injection approach or the setter injection approach, both of which promote good coding practices such as separation of concerns, testability, and modularity.
5- Dependency Injection Annotations
When working with Dependency Injection in Java, there are various annotations that you can use to make your code more readable and maintainable. In this section, we will look at five essential Dependency Injection Annotations that you should be familiar with.
1. `@Inject`
The `@Inject` annotation is the most fundamental Dependency Injection Annotation in Java. It is used to mark a class constructor, method, or field that should be injected with a dependent object. Take a look at the following example:
public class MyService {
private final MyDao myDao;
@Inject
public MyService(MyDao myDao) {
this.myDao = myDao;
}
}
In the above code, the `MyService` constructor is marked with the `@Inject` annotation, indicating that the `MyDao` object should be injected into the constructor.
2. `@Named`
The `@Named` annotation is used to specify the name of the object to be injected. It is used in conjunction with the `@Inject` annotation. Take a look at the following example:
public class MyService {
@Inject
@Named("myDaoImpl")
private MyDao myDao;
}
In the above code, the `@Named` annotation is used to specify that the `MyDao` object to be injected should be of type `myDaoImpl`.
3. `@Singleton`
The `@Singleton` annotation is used to specify that a managed bean should be created as a Singleton. This means that only one instance of the managed bean will be created and shared across the application. Take a look at the following example:
@Singleton
public class MyBean {
// ...
}
In the above code, the `MyBean` class is marked with the `@Singleton` annotation, indicating that only one instance of the `MyBean` class will be created and shared across the application.
4. `@RequestScoped`
The `@RequestScoped` annotation is used to specify that a managed bean should be created and managed for the duration of a single HTTP request. This means that a new instance of the managed bean will be created for each HTTP request. Take a look at the following example:
@RequestScoped
public class MyBean {
// ...
}
6- Constructor Injection vs Setter Injection
In the world of dependency injection, there are two ways to inject dependencies into a class: constructor injection and setter injection. Understanding the main differences between these two approaches is essential for anyone new to the concept of dependency injection.
What is Constructor Injection?
Constructor injection is a technique for injecting dependencies into a class by passing them as constructor parameters. In this approach, the dependencies are provided to the class at the time of its creation, which makes it easy to create immutable objects with all the necessary dependencies.
Advantages of Constructor Injection:
Provides a clear, well-defined interface for dependencies.
Guarantees that all necessary dependencies are available at the time of object creation.
Makes it easy to create immutable objects.
Disadvantages of Constructor Injection:
Can make constructors complex and hard to read.
Can be challenging to inject dependencies that are not all available at the same time.
What is Setter Injection?
Setter injection is a technique for injecting dependencies into a class by providing setter methods. In this approach, the class is first created with default values, and then the dependencies are set using the setter methods.
Advantages of Setter Injection:
Makes it easy to add or remove dependencies at runtime.
Can handle optional dependencies that may not be required for all use cases.
Disadvantages of Setter Injection:
Can lead to code duplication when setters are called multiple times.
Can make it hard to create immutable objects.
Main difference between Constructor Injection and Setter Injection :
Constructor injection ensures all mandatory dependencies are set when an object is created. Setter injection, on the other hand, allows you to set optional dependencies as and when required.
(static field and method)
It is worth noting there is a third type of injection that makes use of static fields and methods. In this approach, dependencies are injected not into instances of a class, but rather into the class itself.
Advantages of static field and method injection:
Can be used to provide global settings across an application.
Allows sharing of dependencies between multiple instances of a class.
Disadvantages of static field and method injection:
Can lead to tight coupling between classes.
Can make it hard to test and maintain code.
💡 key Takeaway: In summary, constructor injection is a great way to ensure that all mandatory dependencies are set when an object is created, whereas setter injection is more flexible and can be used for optional dependencies. It is essential to choose the appropriate technique based on the use case, keeping in
7- Field Injection vs Constructor Injection
When it comes to implementing Dependency Injection in Java, there are two main techniques: field injection and constructor injection. Both methods are used to satisfy dependencies automatically without an object knowing how to create those dependencies. However, each method has its pros and cons, and it's important to understand the differences between them to determine which one is more suitable for your project.
Field injection is a technique where dependencies are set as fields in a class, and the container uses reflection to set the values of those fields with instances of the required dependencies. This method is straightforward to implement and requires little code. However, field injection has some drawbacks that should be considered. One significant disadvantage is the tight coupling of classes that field injection can cause. In case a field is not properly initialized, the container would throw an exception at runtime. Additionally, field injection makes testing harder, as injected dependencies can not be easily swapped for fakes.
On the other hand, constructor injection is a technique in which dependencies are passed to a constructor, which then assigns them to member variables. This method ensures that all necessary object instantiation has taken place before an object in a class is created. Constructor injection makes your code more testable as dependencies can be explicitly passed and tested much easier. The disadvantage of constructor injection is in its verbosity. Developers need to write additional code to create objects and pass their dependencies.
An important note to keep in mind is that while dependency injection is a useful technique, not all dependencies can be satisfied through it. In some cases, a class might require a hard dependency that must be present at compile time. In such cases, DI patterns won't work, and the developer should instead use a lower-level class.
💡 key Takeaway: Determining which dependency injection approach to use in your Java project, whether field injection or constructor injection, is essential when it comes to ensuring a program's quality, simplicity, and testability.
8- Advanced Dependency Injection Techniques
In addition to the basic dependency injection techniques, there are a number of more advanced techniques that Java developers can use to effectively manage dependencies in their code. Here are some of the most useful advanced dependency injection techniques:
1. Setter Injection
Setter injection is a technique where an object's dependencies are set by calling setter methods on the object after it has been constructed. This allows for greater flexibility and easier testing of the object since its dependencies can be easily swapped out.
2. Constructor Injection
Constructor injection is a technique where an object's dependencies are provided as constructor arguments when the object is constructed. This is a more static approach to injecting dependencies, but it can be beneficial in situations where certain dependencies are required for the object to function properly.
3. Interface Injection
Interface injection is a technique where an object implements an interface that defines how dependencies should be injected. This can be useful in situations where an object needs to work with different types of dependencies at runtime.
4. Automatic Injection
Automatic injection is a technique where dependencies are automatically injected into an object without any explicit configuration. This is typically done using a framework or container that is responsible for managing dependencies.
5. Stop Hook Injection
Stop hook injection is a technique where an object provides a method that is called when the object is no longer needed. This allows for cleanup to be performed before the object is destroyed.
6. Service Locator Pattern
The service locator pattern is a technique where a centralized registry is used to manage dependencies. This registry can be used to lookup and retrieve dependencies as needed, allowing for greater flexibility and decoupling of objects.
7. Contextualized Dependency Injection
Contextualized dependency injection is a technique where dependencies are injected based on the context in which the object is being used. This can be useful in situations where different dependencies are required based on the environment or other factors.
8. Message / Event Driven Injection
Message/event-driven injection is a technique where dependencies are injected in response to messages or events. This allows for greater flexibility and dynamic control over dependencies.
💡 key Takeaway: By using advanced dependency injection techniques such as setter injection, constructor injection, interface injection, automatic injection, stop hook injection, service locator pattern, contextualized dependency injection, and message/event-driven injection, Java developers can effectively manage dependencies in their code, resulting in greater flexibility and easier testing.
Conclusion
In conclusion, understanding dependency injection is crucial for Java developers who want to build scalable and maintainable applications. By implementing this design pattern, you can reduce the complexity of your code, promote loose coupling between classes, and facilitate unit testing. With some practice and experimentation, you can become proficient in dependency injection and accelerate your Java programming skills. So, whether you are a beginner or an experienced developer, take the time to master this powerful technique and see the benefits in your projects. Don't forget to apply these principles to your next Java project and share your feedback with us in the comments section below. Start implementing these tips today and take your Java programming skills to the next level!
FAQ
What are the different dependency injection frameworks?
There are several dependency injection frameworks available, but the two most popular are Spring and JavaBean.
What are the different ways to inject dependencies into fields?
There are several ways to inject dependencies into fields. The most common way is to use the constructor parameter. You can also inject dependencies through annotations.
What are the different ways
There are different ways of injecting dependencies in Java code. This can be done through constructor injection, property injection, or interface injection.
What is dependency injection?
Dependency injection is a design pattern that allows an object to be created without knowing all of its dependencies. This is useful when you want to decouple an object’s dependency on other objects.
How do I use dependency injection in my Java code?
In this article, we will discuss dependency injection and show you how to use it in your Java applications. Dependency injection is a technique that allows you to inject the dependencies of an object into another object without having to specify those dependencies explicitly.
What are the benefits of dependency injection?
One of the advantages of dependency injection is that it makes it much easier to configure your objects. This is because you can inject the dependencies into the object instead of having to pass them as arguments. This makes it much easier to test your code.
What is the difference between constructor injection and setter injection?
Constructor injection is a technique that allows you to inject an object into a constructor. This is done by specifying the constructor name as the argument to the injection point. Setter injection is a technique that allows you to inject an object into a setter. This is done by specifying the setter name as the argument to the injection point.
What are the different ways to inject dependencies into my code?
There are several ways to inject dependencies into your code: By using the @Inject annotation: @Inject private Dependency dependency; By using the Dagger 2 annotation processor: @Inject private Dagger dependency; By using the constructor injection syntax: new Dependency(...
What are the different types of dependency injection?
There are two main types of dependency injection: constructor dependency injection and class dependency injection. With constructor dependency injection, you inject dependencies into the constructor of a class. This is the simplest form of dependency injection and is used when you don’t need to inject any dependencies into any other methods in the class. With class dependency injection, you inject dependencies into the class itself. This is more powerful than constructor dependency injection because you can inject dependencies into any method in the class.
Dependency injection is an increasingly popular design pattern used in Java programming. It's an essential tool for organizing code and achieving greater efficiency in software development. With dependency injection, you can more easily maintain code, create re-usable components, and work confidently with dynamic databases.
In this guide, we'll look at the basics of dependency injection and its applications in Java. We'll cover the fundamentals of dependency injection from registering services to retrieving dependencies from a container. By the end of this guide, you should understand the core concepts of dependency injection and be able to apply it to your own projects.